As web developers, we closely monitor the shifts in today’s modern web applications architecture stack. We find the client vs. server-side HTML rendering debate particularly interesting.
In the past several years, we’ve witnessed the enormous rise in popularity of client-side JS/CoffeeScript MVC & MVVM solutions. From popular libraries such as Backbone.js that strive to add basic structure to client-side apps, all the way to feature-rich libraries that manage your entire client-side stack, with data-binding, client-server model sync, dependency tracking, templates and more. The involvement of high-profile companies and individuals in this market is also fascinating, between KnockoutJS contributions from Microsoft, the Google-backed Angular and Yehuda Katz’s Ember, the heat is definitely on.
Client-side rendering adoption will most likely continue to grow as major companies adopt the concept and treat the browser as just another device to access their server-based APIs. Twitter is one of these companies. Twitter’s engineers has moved to client-side rendering a year and a half ago as part of their last major redesign work. They even went a step further and open sourced their entire Javscript toolbelt as part of the Bootstrap project.
Since we at Cloudinary have been longtime fans of server-side rendering, the first web application development framework we’ve integrated Cloudinary with was Ruby-on-Rails.
Due to popular demand, we really wanted to help simplify image management for Javascript developers – client-side rendering and node.js developers alike. Fortunately, Cloudinary’s techniques are already based on simple URL and HTTP interfaces that can be easily integrated with any web-dev framework.
Without further ado, we want to introduce Cloudinary’s jQuery plugin. It is open-source and available at GitHub.
We have big plans for this jQuery plugin. We’ll tell you more about it in the follow-up posts. In the meantime, we wanted to show you how to easily integrate Cloudinary for embedding images and transforming images using Javascript.
For example, a JPG file was uploaded to Cloudinary and was assigned the ‘sample’ public ID.
First, configure your cloud name:
$.cloudinary.config("cloud_name", "your_cloud_name_goes_here");
The following Javascript command returns the URL of a 150×100 transformed version of the uploaded image:
$.cloudinary.url("sample.jpg", { width: 150, height: 100, crop: 'fill', gravity: 'south'});
It returns the following Cloudinary URL that goes through AWS CloudFront CDN. Note that ‘demo’ should be replaced with your Cloudinary’s cloud name.
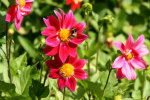
Cloudinary’s jQuery plugin includes the ‘image‘ function. It returns an IMG HTML element that you can add to your page.
The following example generates a 150×100 PNG version of the uploaded image, this time with rounded corners. It also generated an IMG tag and adds it to the HTML DOM elements with the ‘image_holder‘ class:
$('.image_holder').append( $.cloudinary.image("sample.png", { width: 150, height: 100, crop: 'fill', gravity: 'south', radius: 20} ).addClass('my_class_name'));
This would generate the following HTML elements:
<div class="image_holder"> ... <img width="150" height="100" class="my_class_name" src="https://res.cloudinary.com/demo/image/upload/ w_150,h_100,c_fill,g_south,r_20/sample.jpg"/> </div>
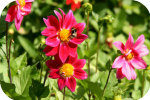
You can use the same method to create an image tag with a Facebook profile picture. Simply specify the user’s Facebook name, fan page name or numeric ID and the required format.
For example, the following Javascript commands returns Cristiano Ronaldo’s Facebook profile picture. Using Cloudinary’s image transformations, the picture is cropped to a face detection based thumbnail of 80×100 pixels.
$.cloudinary.facebook_profile_image("Cristiano.jpg", { width: 80, height: 100, crop: 'thumb', gravity: 'face'});
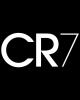
Note: ‘$‘ in all examples can be replaced with ‘jQuery’.
Another useful thing you can do with the jQuery plugin is lazy loading of images and resizing on demand and on the fly.
For example, add the following image tag to your HTML:
<img src="blank.png" class="dynamic_image”" data-src="couple.jpg" data-width="115" data-height="135 data-crop="thumb" data-gravity="faces" data-radius="20"/>
It points to a placeholder ‘blank.png’ image, but the actual image is ‘couple.jpg’. ‘couple’ is a public ID of an image previously uploaded to Cloudinary. All attributes starting with ‘data-‘ are parameters to Cloudinary’s image transformation engine.
Simply running ‘cloudinary’ on a given jQuery selector would process all images matching the given selector. The following example processes all elements with the dynamic_image class when the document is ready:
$(document).ready(function() { $(".dynamic_image").cloudinary(); });
This would automatically generate transformed versions on the fly. In this example, a 115×135 rounded-cornered thumbnail based on face detection. The transformed image is delivered through a fast CDN. The IMG tag would be converted to the following and the requested image would be displayed:
<img src="https://res.cloudinary.com/demo/image/upload/ w_115,h_135,c_thumb,g_faces,r_20/couple.jpg" width="115" height="135" class="dynamic_image"/>
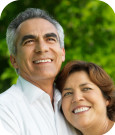
What do you think? We would love to hear your feedback on our jQuery plugin and your ideas for enhancements. We are already working on some cool additions, we would update you soon…