Python SDK
Last updated: Apr-09-2024
This page provides an in-depth introduction to the Python SDK.
We invite you to try the free Introduction to Cloudinary for Python Developers online course, where you can learn how to upload, manage, transform and optimize your digital assets.
Overview
Cloudinary's Python SDK provides simple, yet comprehensive image and video upload, transformation, optimization, and delivery capabilities that you can implement using code that integrates seamlessly with your existing Python application.
For details on all new features and fixes from previous versions, see the CHANGELOG.
Quick example: Transformations
Take a look at the following transformation code and the image it delivers:
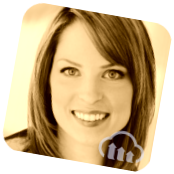
This relatively simple code performs all of the following on the original front_face.jpg image before delivering it:
- Crop to a 150x150 thumbnail using face-detection gravity to automatically determine the location for the crop
- Round the corners with a 20 pixel radius
- Apply a sepia effect
- Overlay the Cloudinary logo on the southeast corner of the image (with a slight offset). The logo is scaled down to a 50 pixel width, with increased brightness and partial transparency (opacity = 60%)
- Rotate the resulting image (including the overlay) by 10 degrees
- Convert and deliver the image in PNG format (the originally uploaded image was a JPG)
And here's the URL that would be included in the image tag that's automatically generated from the above code:
In a similar way, you can transform a video.
- See all possible transformations in the Transformation URL API reference.
- See more examples of image and video transformations using the Cloudinary Python library.
Quick example: File upload
The following Python code uploads the dog.mp4
video to the sub-folder path, myfolder/mysubfolder
. The resulting public_id will be myfolder/mysubfolder/dog
. The video will overwrite the existing video if a video with that public_id already exists. When the video upload is complete, the specified notification URL will receive details about the uploaded media asset.
Python Library features
Cross-framework features (Flask & Django)
- Build URLs for image and video transformation
- API wrappers: file upload, administration, sprite generation and more
- Server-side file upload + direct unsigned file upload from the browser using the jQuery plugin
Django-specific features
- Form and model integration classes
- Template tags for embedding and transforming resources
Installation
Cloudinary's Python integration library is available as open-source Python code.
-
Install Cloudinary's module using either easy_install or pip package management tools:
Add
cloudinary
to the list of INSTALLED_APPS in settings.py.-
Include Cloudinary's Python classes in your code:
{% load cloudinary %}
Configuration
To use the Cloudinary Python library, you have to configure at least your cloud_name
. Your api_key
and api_secret
are also needed for secure API calls to Cloudinary (e.g., image and video uploads). You can find your product environment configuration credentials in the Dashboard page of the Cloudinary Console.
In addition to the required configuration parameters, you can define a number of optional configuration parameters if relevant.
Setting the configuration parameters can be done globally, using either an environment variable or the config
method, or programmatically in each call to a Cloudinary method. Parameters set in a call to a Cloudinary method override globally set parameters.
secure
configuration parameter is false
. However, for most modern applications, it's recommended to configure the secure
parameter to true
to ensure that your transformation URLs are always generated as HTTPS.Setting the CLOUDINARY_URL environment variable
You can configure the required cloud_name
, api_key
, and api_secret
by defining the CLOUDINARY_URL environment variable. The CLOUDINARY_URL value is available in the Dashboard page of the Cloudinary Console. When using Cloudinary through a PaaS add-on (e.g., Heroku or AppFog), this environment variable is automatically defined in your deployment environment. For example:
Set additional parameters, for example upload_prefix and cname, to the environment variable:
Setting configuration parameters globally
Here's an example of setting configuration parameters globally in your Python application:
cloudinary.uploader
and cloudinary.api
classes in order to pass the optional api_proxy configuration parameter successfully:
Python configuration and installation video tutorial
Watch this video tutorial to see how to install and configure the Python SDK:
Tutorial contents
Python capitalization and data type guidelines
When using the Python SDK, keep these guidelines in mind:
- Parameter names:
snake_case
. For example: public_id - Classes:
PascalCase
. For example: CloudinaryField - Methods:
snake_case
. For example: add_tag - Pass parameter data as:
dict or named arguments
Full Flask demo app and code sandbox
This demo uses Cloudinary's auto-tagging feature to recommend product images based on the images you select. Try out the demo and then check out the code behind it.
This code is also available in GitHub.
- Read this blog to discover all the Cloudinary features in this demo.
Jupyter notebook
This Jupyter notebook focuses on uploading images to your product environment. It walks you through:
- Installing and configuring Cloudinary.
- Creating an upload preset.
- Applying the preset to assets being uploaded.
- Uploading assets with incoming transformations.
To run the code snippets in the Jupyter notebook, you'll need to enter your credentials as part of configuration. Find your credentials in the Dashboard page of your Cloudinary Console.
Sample projects
For additional useful code samples and learn how to integrate Cloudinary with your Python applications, take a look at our Sample Projects.
- Basic python sample: Uploading local and remote images to Cloudinary and generating various transformation URLs.
- Basic Flask - image uploader and transformer: Uploading images to Cloudinary and displaying them on a webpage with various transformations applied.
- Django photo album: A fully working web application that allows you to upload photos, maintain a database with references, list images with their metadata, and display them using various cloud-based transformations. Image uploading is performed both from the server side and directly from the browser using a jQuery plugin.
- Try out the Python SDK using the quick start.
- Learn more about uploading images and videos using the Python SDK.
- See examples of powerful image and video transformations using Python code
and see our image transformations and video transformation docs.
- Check out Cloudinary's asset management capabilities, for example, renaming and deleting assets, adding tags and metadata to assets, and searching for assets.
- Stay tuned for updates with the Programmable Media Release Notes and the Cloudinary Blog.