Shopping online is convenient, but customers often miss being able to physically interact with products in brick-and-mortar stores. In fact, 85% of shoppers favor the in-store experience. Without this information, it can be challenging to make a purchase decision.
One way to replicate the in-store experience is to create immersive 3D image models that provide your customers with an interactive, realistic view of the product. This technology can be a real differentiator between an abandoned cart and a sale. According to a Cloudinary survey, 53% of respondents said they were more likely to buy if they had access to 3D product images.
This blog post will show you how to use Cloudinary’s Dimensions product to embed 3D models in an e-commerce website using Next.js.
Creating a 3D model can be challenging, but fortunately, specialized software is available to make the process easier. For example, Polycam is an app for your phone that lets you take shots of an object from different angles and then produce a 3D model. This app fits into the so-called photogrammetry software category, which analyzes images by identifying common points in each one and connecting them to create a 3D view.
We’ll work with Polycam for this blog post, but any other photogrammetry software will work.
After installing and opening the app, click the camera icon to make a new capture. You’ll be offered two capture modes: LiDAR and Photo. The LiDAR mode is used to capture larger areas, such as rooms or apartments. On the other hand, the Photo mode is usually the best choice for capturing objects where accuracy and detail are essential, and this is the mode we’ll be using. This mode works by taking a sequence of photos and uploading them to the cloud, where they’re processed to create a 3D image reconstruction.
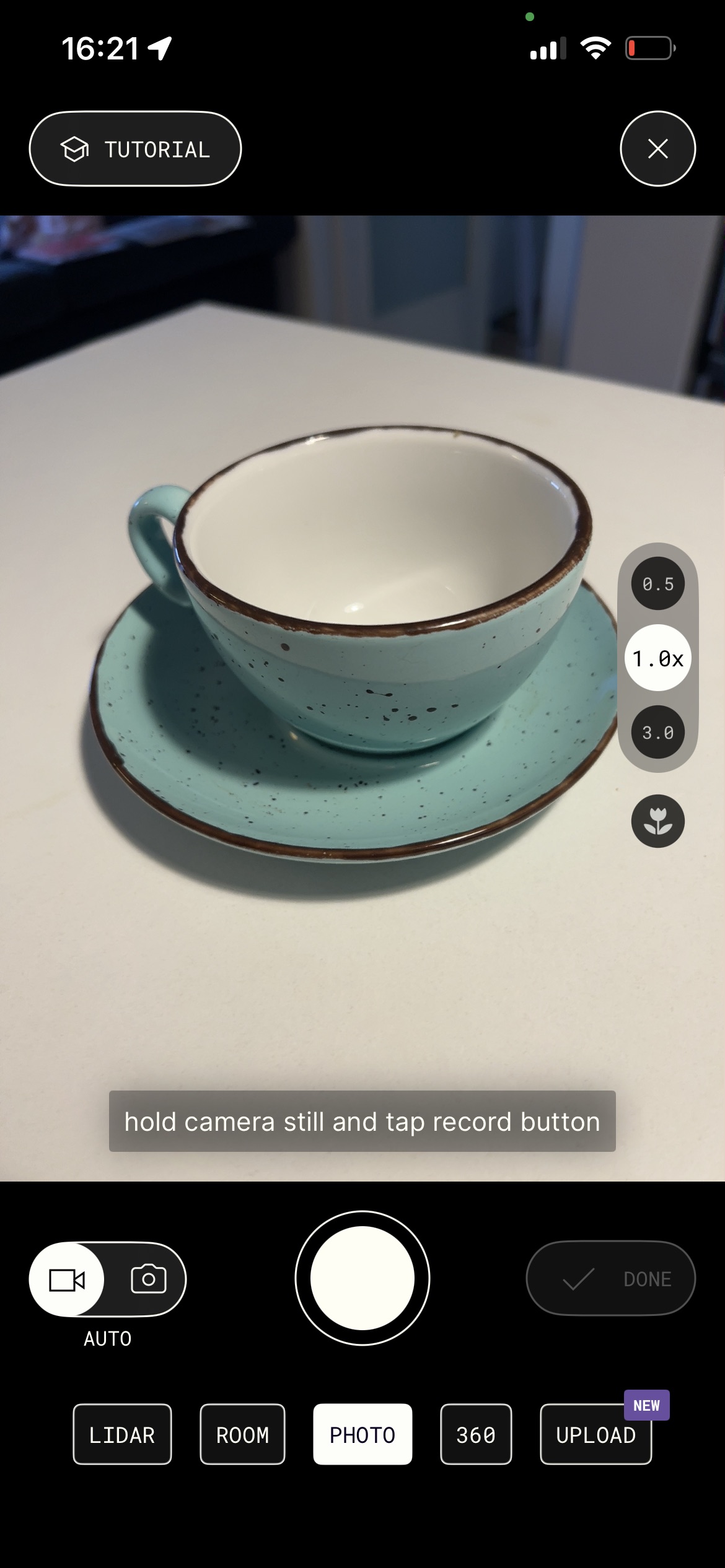
Press the Record button and take photos of the object you want to create a 3D model of. Once you’re done, click Record again, which will upload the pictures to the cloud for processing. Once the processing is complete, you can click the image and then the Download icon in the top-right corner.
You’ll be given several export options; the one we’re interested in is the GLTF format. This format is specifically designed for fast transmission and use of 3D models in web browsers and VR environments. After clicking GLTF, you’ll receive a ZIP file containing a GLB file that represents your 3D model.
Dimensions is a new product from Cloudinary, currently in beta, allowing you to generate assets from your 3D models and deliver them through the Cloudinary API. To use it, log in to the Dimensions Catalog and click Upload 3D Model to upload the previously saved GLB file.
As you can see, our 3D model has room for improvement, and there are certainly far better-quality models out there, but we’re using it just to demonstrate how it can be used in Dimensions. To get a higher-quality model, you’d need to have a different environment with better lighting so that the actual object can be isolated from the background.
Next, click Create a 3D Viewer and choose one of the available sizes. Then click View Results, and the model will be uploaded and made available in a 3D viewer. We can now use this 3D model in our e-commerce website, which we’ll implement next.
Before proceeding, note the “SKU” value displayed at the top of the page. We’ll need this later.
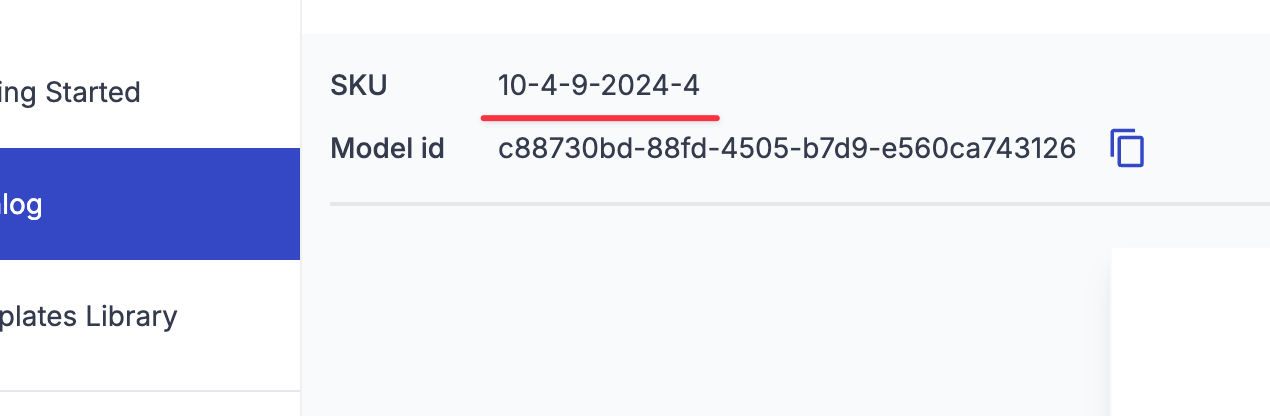
The first step is to go to the Dimensions Settings page and note down the value of the Account Name field. We’ll need to store this value as an environment variable for use by Next.js.
When running locally, this will be done in the .env.local
file:
NEXT_PUBLIC_CLOUDINARY_ACCOUNT_NAME=[your account name]
The environment variable is prefixed with NEXT_PUBLIC_
because it’s an identifier that’s safe to be used in the browser context.
Next, define a component responsible for including the necessary Dimensions JavaScript libraries. These libraries are required for initializing the Dimensions client and parsing custom HTML attributes that Dimensions uses.
You can see the source code for the whole component below:
// components/dimensions-script.tsx
"use client";
import Script from "next/script";
import { useEffect } from "react";
const CLOUDINARY_ACCOUNT_ID = process.env.NEXT_PUBLIC_CLOUDINARY_ACCOUNT_NAME;
export default function DimensionsScript() {
useEffect(() => {
// @ts-expect-error type definition initDimensions is not yet available
window.initDimensions({
account: CLOUDINARY_ACCOUNT_ID,
viewers: ["3D"],
});
console.log(
"Cloudinary Dimensions initialized for account",
CLOUDINARY_ACCOUNT_ID,
);
}, []);
return (
<>
<Script
strategy="beforeInteractive"
src="https://dimensions-tag.cloudinary.com/all.js"
/>
);
}
Code language: JavaScript (javascript)
This component adds the base Dimensions library necessary for initialization. To ensure this happens as soon as possible, it’s executed using the beforeInteractive
strategy. The other script is used to render the actual 3D viewer.
The component also runs on the initDimensions
function provided by the first JavaScript library when mounted. For this reason, it needs to be a client component, as noted by the use client
directive at the top.
// app/layout.tsx
export default function RootLayout({
children,
}: Readonly<{
children: React.ReactNode;
}>) {
return (
<html lang="en">
<body>
{children}
<DimensionsScript />
</body>
</html>
);
}
Code language: JavaScript (javascript)
With the Dimensions library initialized, we can use Dimensions’ custom HTML tags anywhere in the site. For example, on a product page. Assuming we have a ProductDetail
component, all we need to do is include a div
element with Dimensions’ custom HTML tags:
export function ProductDetail() {
const skuId = "sneaker-123";
return (
<div className="grid md:grid-cols-2 gap-6 lg:gap-12 items-start max-w-6xl px-4 mx-auto py-6">
<div className="grid gap-4 md:gap-10 items-start h-full">
<div className="grid gap-4 h-full">
{/* This will embed the 3D model */}
<div data-d8s-type="3d" data-d8s-id={skuId}></div>
</div>
</div>
<div className="grid gap-4 md:gap-10 items-start">
{/* The rest of the product detail page */}
</div>
</div>
);
}
Code language: JavaScript (javascript)
Here, the data-d8s-type
attribute is set to 3d
to render the Dimensions viewer with the model associated with the product. The data-d8s-id
attribute refers to the SKU. The data-d8s-typ
e attribute can also be set to video
, or it defaults to image
if not set explicitly. With this code in place, you should now see an animated 3D image of our product.
Cloudinary Dimensions is a powerful product to help you make immersive 3D models with ease and at scale. All you need to do is upload your model and embed it in your app using the Cloudinary libraries. Although it’s still in beta, you’re free to try it out at the official Dimensions website.