Android image transformations
Last updated: Oct-31-2023
Overview
After you or your users have uploaded image assets to Cloudinary, you can deliver them via dynamic URLs. You can include instructions in your dynamic URLs that tell Cloudinary to transform your assets using a set of transformation parameters. All transformations are performed automatically in the cloud and your transformed assets are automatically optimized before they are routed through a fast CDN to the end user for optimal user experience.
For example, you can resize and crop, add overlay images, blur or pixelate faces, apply a large variety of special effects and filters, and apply settings to optimize your images and to deliver them responsively.
Cloudinary's Android library simplifies the generation of transformation URLs, for easy embedding of assets in your Android application.
See also: Android video transformation
Generate image URLs
Use the url
method of the MediaManager class to generate an image URL.
The code above returns the following string:
https://res.cloudinary.com/demo/image/upload/sample.jpg
Add Transformations
You can add transformations to your url
method by defining them with the Transformation
class and then applying them with the transformation
method of the MediaManager class.
For example:
Chaining Transformations
You can also chain transformations together (each transformation is applied to the result of the previous transformation):
For example, the following code crops the image to 150x150, rounds the corners, applies a sepia effect, adds text to the top center of the resized image, and then rotates the entire result by 20 degrees.
For more information and examples of image transformations, see Applying common image transformations.
Download images
The dynamic URL returned from the url
method can be easily used with most of the popular download libraries. For example:
Cloudinary also supports 2 other options for downloading images with a download library:
- Glide integration module - an extension for Glide
- Cloudinary download adapter - an adapter for download libraries
Glide integration module
The Cloudinary Glide integration module allows you to integrate Cloudinary functionality within a Glide image download by passing a cloudinaryRequest
directly to the Glide image-download API. The cloudinaryRequest
is embedded directly within the code for a Glide download, and provides a means to send Cloudinary transformation and responsive requests seamlessly, asynchronously, and in easy-to-follow code.
Installing the module
To add the Cloudinary Glide integration module, add this dependency to your build.gradle
file:
If you don't already have a class extending from AppGlideModule, you'll need to create one, and make sure it's annotated as follows (there's no need for any code in the body of the class):
Using the module
The flow is identical to a regular Glide download, but instead of sending a URL or a bitmap, you can send an instance of a CloudinaryRequest
. You can use a builder to construct an instance of a CloudinaryRequest
without the need to generate a full URL. The builder must include the public_id
of the image and also supports Transformations and ResponsiveUrls (or a responsive Preset as a shorthand). The download URL is constructed internally, injecting any runtime view dimensions as required, and downloads the Cloudinary asset into the image view through the standard Glide pipeline.
For example, to deliver the 'sample' image with a blur effect applied, and automatically fill the available view dimensions:
Cloudinary download adapter
The Cloudinary download adapter provides built-in integration for the three major image download libraries in the Android ecosystem - Glide, Fresco and Picasso, although you can also integrate any 3rd party download library of your choice with very little effort. The adapter wraps an existing 3rd party download library already in use, with support for directly using public ids, transformations and client-side responsiveness. All the downloaded URLs are transformed as required, and then delegated to the underlying download library.
Installing the adapter
To add the Cloudinary download adapter:
-
Add the following dependency to your
build.gradle
file: -
If you haven't already added a download library, do so as follows:
-
Set the appropriate adapter for your initialized MediaManager, based on your selected download library:
Using the adapter
The functionality is available through the MediaManager entry point by calling MediaManager.get().download(context)
, which returns a request builder. There are two methods that must be called to get a remote image loaded into an image view:
-
load
- selects what to load into the image view:-
resource
- an Integer representing an android resource id. -
source
- a string representing either a remote URL or a Cloudinary public id.
-
-
into
- starts that download process into the given ImageView instance.
In addition to these two methods there are 4 optional setters for the builder, allowing for further customization of the downloaded asset:
setter | type | description |
---|---|---|
transformation | Transformation | A transformation to apply to the public id. |
responsive | ResponsiveUrl or a ResponsiveUrl.Preset | Automatically adjust the size of the image according to the view's dimensions. Either: FIT , AUTO_FILL or MediaManager.get().responsiveUrl({autoWidth}, {autoHeight}, {cropMode}, {gravity}) . See Responsive images for more details. |
callback | DownloadRequestCallback | Called once the image has finished downloading, or if the request frayed. |
placeholder | Int (android resource id). | A placeholder to use in the ImageView. |
transformation
and responsive
are only relevant when calling load
with a Cloudinary public id.For example:
Thanks for your time!
Apply common image transformations
This section provides an overview and examples of the following commonly used image transformation features, along with links to more detailed documentation on these features:
- Resizing and cropping
- Converting to another image format
- Applying image effects and filters
- Adding text and image overlays
- Image optimizations
Keep in mind that this section is only intended to introduce you to the basics of using image transformations with Android.
For comprehensive explanations of how to implement a wide variety of transformations, see Image transformations. For a full list of all supported image transformations and their usage, see the Transformation URL API Reference.
Resizing and cropping
There are a variety of different ways to resize and/or crop your images, and to control the area of the image that is preserved during a crop.
The following example uses the fill
cropping method to generate and deliver an image that completely fills the requested 250x250 size while retaining the original aspect ratio. It uses face detection gravity to ensure that all the faces in the image are retained and centered when the image is cropped:
You can also use automatic gravity to determine what to keep in the crop automatically.
For details on all resizing and cropping options, see resizing and cropping images.
Converting to another image format
You can deliver any image uploaded to Cloudinary in essentially any image format. There are two main ways to convert and deliver in another format:
- Specify the image's public ID with the desired extension.
- Explicitly set the desired format using the
fetchFormat
parameter.
For example:
Deliver a .jpg file in .gif format by specifying the new file extension:
Deliver a .jpg file in .gif format by specifying the fetchFormat
parameter:
For more details see Image format conversion.
Applying image effects and filters
You can select from a large selection of image effects, enhancements, and filters to apply to your images. The available effects include a variety of color balance and level effects, tinting, blurring, pixelating, sharpening, automatic improvement effects, artistic filters, image and text overlays, distortion and shape changing effects, outlines, backgrounds, shadows, and more.
For example, the code below applies a cartoonify effect, rounding corners effect, and background color effect (and then scales the image down to a height of 300 pixels).
For more details on the available image effects and filters, see Visual image effects and enhancements.
Adding text and image overlays
You can add images and text as overlays on your main image. You can apply the same types of transformations on your overlay images as you can with any image and you can use gravity settings or x and y coordinates to control the location of the overlays. You can also apply a variety of transformations on text, such as color, font, size, rotation, and more.
For example, the code below overlays a couple's photo on a mug image. The overlay photo is cropped using face detection with adjusted color saturation and a vignette effect applied. The word love is added in a pink, fancy font and rotated to fit the design. A balloon graphic is also added. Additionally, the final image is cropped and the corners are rounded.
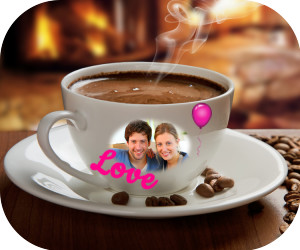
Image optimizations
By default, Cloudinary automatically performs certain optimizations on all transformed images. There are also a number of additional features that enable you to further optimize the images you use in your Android application. These include optimizations to image quality, format, and size, among others.
For example, you can use the auto
value for the fetchFormat
and quality
attributes to automatically deliver the image in the format and quality that minimize file size while meeting the required quality level. Below, these two parameters are applied, resulting in a 50% file size reduction (1.4 MB vs. 784 KB) with no visible change in quality.
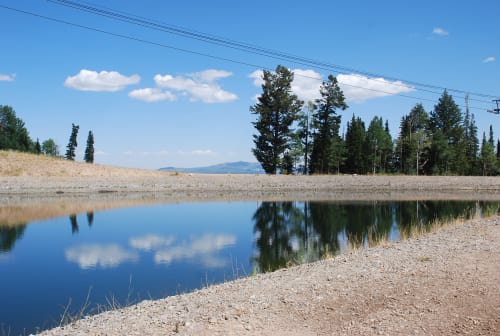
For an in-depth review of the many ways you can optimize your images, see Image optimization.
Responsive images
Responsive design is a method of providing an optimal viewing experience to users, tailored to their device, viewport size, orientation, and resolution. An app that is designed responsively adapts its layout to the viewing environment, resizing and moving elements dynamically and based on the properties of the device where the app is displayed.
When it comes to images, a responsively designed app should not just send the highest resolution image and then use client-side resizing to display the image on the various devices: that would be a significant waste of bandwidth for users on small, low-resolution displays. The best solution is to prepare an image in various resolutions and sizes, and then deliver the image with the most appropriate resolution, based on the device's resolution and the available dimensions to avoid wasting bandwidth or load time. Cloudinary can help reduce the complexity of this task with dynamic image transformations. You can simply build image URLs with any image width and height based on the specific device resolution and viewport size. This means you don't have to pre-create the images; the dynamic resizing takes place on the fly as needed.
Use the MediaManager's responsiveUrl
method to generate a dynamic image URL based on the exact dimensions needed for the image according to a specified ImageView
element. In addition to the image's PublicId, you then pass the ImageView
element and a ResponsiveUrl.Callback()
to the generate
method. After the image URL for the specified ImageView size has been generated, you can use any image download library to fetch the image and update the element.
When the responsiveUrl
method runs, it retrieves the exact available dimensions for the ImageView element and then rounds up the height and width for the image to the nearest step (100 by default). For example, if the exact width of the ImageView element is 284 pixels, the requested width is rounded up to 300 pixels. This prevents too many image versions being generated and reduces cache hits for subsequent requests from other devices. The next time any image view requiring 201-300 pixels requests the same image, the existing one is delivered directly from the CDN cache.
The responsiveUrl
method accepts 4 parameters as follows:
-
autoWidth
: Boolean - Adjust image width according to the available ImageView width -
autoHeight
: Boolean - Adjust image height according to the available ImageWidth height -
cropMode
: String - The crop mode to apply when adjusting the image -
gravity
: String - The location in the image to be used as the focus for the transformation
Instead of specifying the 4 parameters individually, you can also pass one of the following presets:
-
AUTO_FILL
: Adjusts both height and width of the image, and crops the image to fill the ImageView while retaining the aspect-ratio and using automatic gravity to determine which part of the image is kept and which is cropped, if necessary. (This is a shortcut for: true, true, "fill", "auto".) -
FIT
: Adjusts both height and width of the image, and resizes the image to completely fit within the bounds of the ImageView while retaining the aspect-ratio. The whole image will be shown. (This is a shortcut for: true, true, "fit", "center".)
For example, to generate the responsiveUrl for the sample
image, automatically resized to fill the available width and height:
Which is equivalent to:
The default values for the responsive calculation can be overridden by calling the stepSize
, minDimension
and/or maxDimension
methods and passing the new values (default values are 100, 100 and 2000 respectively).
For example, to set the step size to every 200 pixels and limit the dimensions to between 200 and 1000 pixels:
Additionally, instead of passing only the PublicId, you can pass any complex Cloudinary dynamic URL, including transformation components. The responsive transformation is chained after everything else as the last component. For example, to generate an HTTPS responsive url, including a transformation to rotate the image by 30 degrees, add a blur effect, and convert the image to WebP: