Conditional image transformations
Last updated: Jan-09-2025
Cloudinary supports conditional transformations for images, where a transformation is only applied if a specified condition is met, for example, if an image's width is greater than 300 pixels, apply a certain transformation.
See also: Conditional transformations for video.
Specifying conditions
To specify a condition to be met before applying a transformation, use the if
parameter (also if
in URLs). The if
parameter accepts a string value detailing the condition to evaluate, and is specified in the URL in the following format:
if_<image characteristic>_<operator>_<image characteristic value>
Where:
-
image characteristic
: The image parameter representing the characteristic to evaluate, for examplew
(orwidth
in SDKs). -
operator
: The comparison operator for the comparison, for examplelt
for 'less than' (or<
in SDKs). -
image characteristic value
: A hard coded value to check against, a supported user-defined variable containing a value to check against, or a different image characteristic you want to compare to.For example, if you only want to apply a transformation to non-square images, you could check if the width characteristic of your image is not equal to its height characteristic:
if_w_ne_h
- Specify strings for a characteristic sub-element or value surrounded by
! !
. For example,if_ if_ctx:!productType!_eq_!shoes!
. - You can check whether a string characteristic currently has no value using
!!
.
For example:if some-condition_eq_!!
See examples below.
Supported image characteristics
Characteristic | Description |
---|---|
w |
(also width in SDKs) The asset's current width. |
iw |
The asset's initial width. |
h |
(also height in SDKs) The asset's current height. |
ih |
The asset's initial height. |
ar |
(also aspect_ratio in SDKs) The aspect ratio of the asset. The compared value can be either decimal (e.g., 1.5) or a ratio (e.g., 3:4). |
iar |
The asset's initial aspect ratio. |
ctx |
A contextual metadata value assigned to an asset. |
md |
A structured metadata value assigned to an asset. |
tags |
The set of tags assigned to the asset. |
tar (trimmed_aspect_ratio in SDKs) |
The aspect ratio of the image IF it was trimmed (using the 'trim' effect) without actually trimming the image. The compared value can be either decimal (e.g., 1.5) or a ratio (e.g., 3:4). |
cp |
The current page in the image/document. |
fc (face_count in SDKs) |
The total number of detected faces in the image. |
rc (regions_count in SDKs) |
The number of named regions set on the image. |
pc (page_count in SDKs) |
The total number of pages in the image/document. |
px |
A layer or page's original x offset position relative to the whole composition (for example, in a PSD or TIFF file). |
py |
A layer or page's original y offset position relative to the whole composition (for example, in a PSD or TIFF file). |
idn |
The initial density (DPI) of the image. |
ils |
The likelihood that the image is an illustration (as opposed to a photo). Supported values: 0 (photo) to 1 (illustration) |
rn (region_names in SDKs) |
The names of regions set on the image. Use with the in or nin operators. |
pgnames |
The names of layers in a TIFF file. Use with the in or nin operators. |
Supported operators
URL | SDK symbol | Description |
---|---|---|
eq |
= |
Equal to |
ne |
!= |
Not equal to |
lt |
< |
Less than |
gt |
> |
Greater than |
lte |
<= |
Less than or equal to |
gte |
>= |
Greater than or equal to |
in |nin
|
in |nin
|
Included in | Not included in Compares a set of strings against another set of strings. See Using the in and nin operators for examples. |
When working with the Cloudinary SDKs, you can specify the condition using the SDK characteristic names and operator symbols, or you can specify it using the URL format. For example, both of the following are valid:
- { if: "w_gt_1000"},...
- { if: "width > 1000"},...
Using the in and nin operators
The in
and nin
operators compare two sets of strings. The :
delimiter between strings denotes AND.
String sets can include tags, contextual metadata or structured metadata values, for example:
- To determine if
sale
andin_stock
are present in the tags of a particular asset, use:if_!sale:in_stock!_in_tags
. - To determine if the key named
color
exists in the contextual metadata of a particular asset, use:if_!color!_in_ctx
. - To determine if a structured metadata field with external ID,
color-id
, has been set for a particular asset, use:if_!color-id!_in_md
. To determine if a list value with external ID,
green-id
, has been selected from a multiple-selection structured metadata field with external ID,colors-id
, for a particular asset, use:if_!green-id!_in_md:!colors-id!
.To determine if a region named
hat
has been set on an image, use:if_!hat!_in_rn
.
For TIFF files:
- To determine if a TIFF file contains a layer called
Shadow
, use:if_!Shadow!_in_pgnames
.
Supported conditional image transformation parameters and flags
-
All image transformation parameters can be assigned in conditions except:
- You cannot assign transformation parameters for the
format
,fetch_format
,default_image
,color_space
, ordelay
parameters. - The
page
(pg
in URLs) parameter cannot be assigned for animated images
(page
can be used in conditions for PSD, PDF, or TIFF documents). - The
angle
parameter cannot be set toignore
.
- You cannot assign transformation parameters for the
Only the following flags are supported inside conditional image transformations:
layer_apply
,region_relative
,relative
,progressive
,cutter
,png8
,attachment
,awebp
,lossy
Notes
- For the
w
,h
,cp
andar
parameters, the values refer to the current image status in the transformation chain (i.e., if transformations have already been applied to the image), whileiw
,ih
,fc
andpc
always refer to the original image. -
dpr
is not supported as a conditional transformation with thecp
andar
characteristics. Additionally,w
andh
are supported withdpr
as long as they are still equal toiw
orih
when the condition is evaluated. Ifdpr
is specified in the transformation as a whole, and one of the conditional branches includes a resizing transformation, you need to specify a resize transformation in all the other branches too. - The
ar
(aspect ratio) parameter should be compared using 'greater than' or 'less than' rather than with 'equals'. This is because the width and height values are given as integers and not floating point values, leading to an "almost exact" calculated aspect ratio. - Contextual metadata values are always stored as strings, even if the value is numeric, therefore you cannot use the
lt
,gt
,lte
andgte
operators to compare contextual metadata values numerically. You can, however, use these operators with numeric structured metadata values - see an example. - You can test whether or not a variable has been defined using the parameters
if_isdef_$<variable name>
andif_isndef_$<variable name>
(see Testing whether a variable has been defined).
Specifying transformations for a condition
The transformation for a condition should be specified between the condition component and an if_end
component in the format:
if_condition/transformation/if_end
For example:
if_ar_lt_1.0/b_auto,c_pad,h_300,w_500/if_end
In the following examples, both images are scaled to a width of 500px. Afterwards, the identical conditional padded-resize transformation shown above is applied to both images. However, since the condition applies only for portrait images (those with an aspect ratio less than 1.0) the resizing and padding is applied only to the mountain-road-boat
image below:
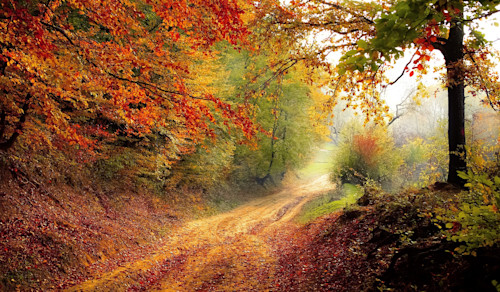
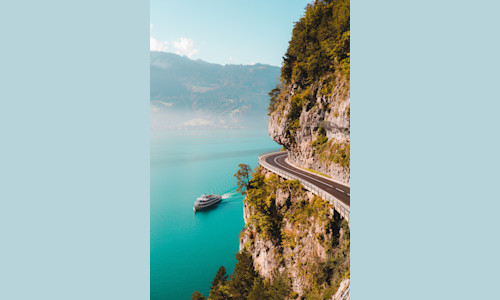
Transformation URLs technically support defining both the condition and a single resulting transformation inside a single transformation component (without the need for a closing end_if
). However, to avoid ambiguity, the best practice is to always use if
and if_end
components in your URL as shown above.
- Some SDKs require this separation and automatically generate URLs in the
if...end_if
format. - A named transformation cannot be placed in the same transformation component as its condition (e.g.,
if_w_eq_h,t_trans
is not supported) and must be specified using theif...if_end
format. - If you do include both the
if
condition and the resulting transformation parameters within a single component of the URL, theif
condition is evaluated first, regardless of its location within the component and (only) when the condition is true, all transformation parameters specified in that component are applied.
Conditions with chained transformations
You can apply multiple chained transformations to your condition by including the entire chained transformation in between the if
and if_end
components.
For example, if you allocate space on your page for an image with a width of 700px, you can conditionally add a 700px width blurred underlay version of the same image along with a text overlay, only for those images whose original width is less than 700px:
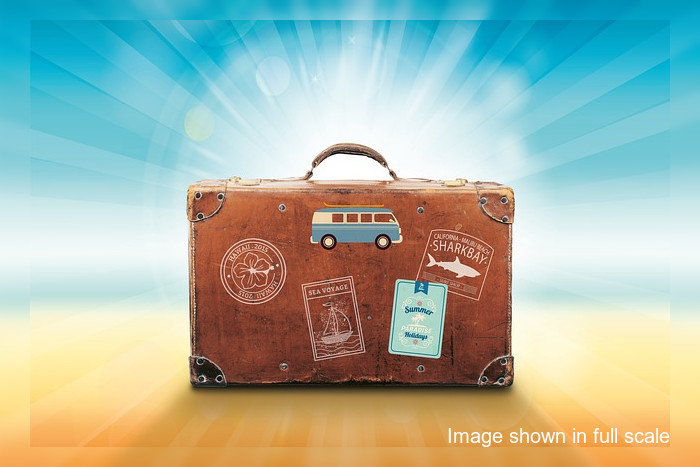
Multiple AND | OR conditions
You can specify multiple conditions to evaluate by joining the conditions with 'AND' or 'OR' conjunction operators.
For example, to crop the an image to a width of 300 pixels and a height of 200 pixels, only if the aspect ratio is greater than 3:4, the width is greater than 300, and the height is greater than 200 (if_ar_gt_3:4_and_w_gt_300_and_h_gt_200
):
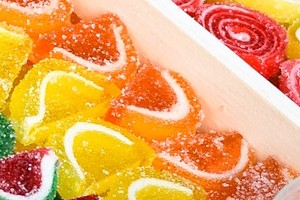
if...end_if
chained components in the URL.Else branch transformations
You can specify a transformation that is applied in the case that the initial condition is evaluated as false (and hence the transformations associated with the condition are not applied), by using the if_else
parameter to specify this fallback transformation.
For example, to set a condition where images with an original width less than or equal to 400px, will be scaled to fill a 240x120px container (if_iw_lte_400/c_fill,h_120,w_240
), while images whose original width is width larger than 400px, will be scaled to fill a 240x400px container (if_else/c_fill,h_240,w_400
):
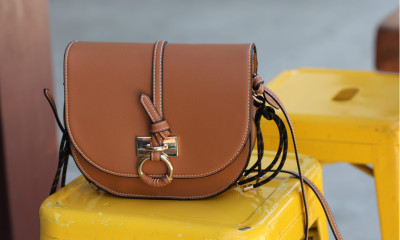
In cases where the if
condition is not in the preceding transformation component, then the if_else
parameter also acts as an if_end
parameter: all chained transformation components until the one with if_else
are only applied if the previous condition holds true. Multiple conditional transformations can also be applied by adding an if_end
parameter to the last transformation component in the chain, and to avoid ambiguity, the component with the if_else
parameter should not have additional transformation instructions.
For example, if the width is less than or equal to 400 pixels then fill the image to 220x180 and add a red effect, else if the width is greater than 400 pixels then fill the image to 190x300 and add an oil painting effect:
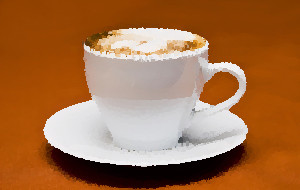
Conditional transformation examples
-
Conditional text overlay based on width: This example limits an image size to a width of 300 pixels using the
limit
crop mode, and then uses a conditional transformation to add a text caption only to images whose initial width was wider than 300 and were scaled down (if_iw_gt_300
): -
Conditional resize based on a contextual metadata value: This example resizes an image to a 200*200 square image if it has a contextual metadata key named 'productType' with the value 'shoes'.
-
Conditional image overlay based on tags: This example adds a sale icon to a product image if both the strings 'sale' and 'in_stock' are among the tags assigned to the image:
-
Conditional image overlay based on structured metadata value: This example adds a sale icon to a product image if the stock level of the product is less than 50 (as determined by the value of the numeric structured metadata field with external ID,
stock-level
, here set as 30):
-
Conditional cropping mode based on illustration score: This example ensures that uploaded graphics such as logos are never cut off, even if the art design significantly changes the required aspect ratio of the delivered image. Using the same transformation, photos can be scaled and cropped to completely fill the full space available.
Using the
ils
conditional characteristic for both URLs below, thecloudinary_icon_blue
logo is resized to the required portrait size to using thepad
method, while theflower_shop
photo is resized to the same aspect ratio using thefill
method:
tags
, ctx
or md
parameters, their values are exposed publicly in the URL. If you want to prevent such values from being exposed, you can disable the Usage of tags/context/metadata in transformation URLs option in the Security Settings (enabled by default). When this setting is disabled, any URL that exposes tags, contextual metadata or structured metadata values will return an error.