Java SDK
Last updated: Apr-30-2025
This page provides an in-depth introduction to the Java SDK.
We invite you to try the free Introduction to Cloudinary for Java Developers online course, where you can learn how to upload, manage, transform and optimize your digital assets.
Overview
Cloudinary's Java SDK provides simple, yet comprehensive image and video upload, transformation, optimization, and delivery capabilities that you can implement using code that integrates seamlessly with your existing Java application.
For details on all new features and fixes from previous versions, see the CHANGELOG.
Quick example: Transformations
Take a look at the following transformation code and the image it delivers:
cloudinary.url().transformation(new Transformation()
.gravity("face").height(150).width(150).crop("thumb").chain()
.radius(20).chain()
.effect("sepia").chain()
.overlay(new Layer().publicId("cloudinary_icon")).chain()
.effect("brightness:90").chain()
.opacity(60).chain()
.width(50).crop("scale").chain()
.flags("layer_apply").gravity("south_east").x(5).y(5).chain()
.angle(10)).secure(true).imageTag("front_face.png");
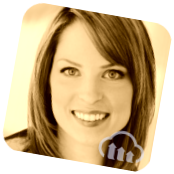
This relatively simple code performs all of the following on the original front_face.jpg image before delivering it:
- Crop to a 150x150 thumbnail using face-detection gravity to automatically determine the location for the crop
- Round the corners with a 20 pixel radius
- Apply a sepia effect
- Overlay the Cloudinary logo on the southeast corner of the image (with a slight offset). The logo is scaled down to a 50 pixel width, with increased brightness and partial transparency (opacity = 60%)
- Rotate the resulting image (including the overlay) by 10 degrees
- Convert and deliver the image in PNG format (the originally uploaded image was a JPG)
And here's the URL that would be included in the image tag that's automatically generated from the above code:
In a similar way, you can transform a video.
- See all possible transformations in the Transformation URL API reference.
- See more examples of image and video transformations using the Cloudinary Java library.
Quick example: File upload
The following Java code uploads the dog.mp4
video using the public_id, my_dog
. The video will overwrite the existing my_dog
video if it exists. When the video upload is complete, the specified notification URL will receive details about the uploaded media asset.
Map params = ObjectUtils.asMap(
"public_id", "my_dog",
"overwrite", true,
"notification_url", "https://mysite.com/notify_endpoint",
"resource_type", "video"
);
Map uploadResult = cloudinary.uploader().upload(new File("doc.mp4"), params);
Java library features
Cloudinary provides an open source Java library for further simplifying the integration:
- Build URLs for image and video transformation
- API wrappers: file upload, administration, sprite generation and more
- JSP tag library to ease and facilitate the inclusion, transformation, upload, and storage in a Java EE web application
- Server-side file upload + direct unsigned file upload from the browser using the jQuery plugin
The library is built for Java 8 / JSP 2.0 and will work with higher versions. The following are resources which serve as a good starting point to better familiarize yourself with the library:
- Full source code under MIT license with a large set of tests.
- Web application samples written for Spring MVC Framework 3.2.
- A Maven package.
Choosing the right Maven package
The Maven repository includes several packages ("artifacts"):
- cloudinary-http - for general Java applications. It utilizes the Apache HTTP libraries, when working with SDK versions v2.x, only HTTP 5 is available.
- cloudinary-taglib - provides a Java Tag Library for J2EE applications
- cloudinary-android - provides support for android applications
Installation and setup
The easiest way to start using Cloudinary's Java library is to use Maven.
- Download and install Maven. Follow https://maven.apache.org/download.cgi for reference.
- Create a maven project. See example here.
-
Add the Cloudinary dependency to the list of dependencies in the pom.xml:
Xml<dependencies> ... <dependency> <groupId>com.cloudinary</groupId> <artifactId>cloudinary-http5</artifactId> <version>[Cloudinary Java SDK version, e.g. 2.0.0]</version> </dependency> </dependencies>
-
If you are building a Java EE web application you should consider using the tag library by adding:
Xml<dependencies> ... <dependency> <groupId>com.cloudinary</groupId> <artifactId>cloudinary-taglib</artifactId> <version>[Cloudinary Java SDK version, e.g. 2.0.0]</version> </dependency> </dependencies>
When using in Java code import the appropriate package:
import com.cloudinary.*;
When using in a JSP view import the tag library:
<%@taglib uri="http://cloudinary.com/jsp/taglib" prefix="cl" %>
Configuration
To use the Cloudinary Java library, you have to configure at least your cloud_name
. An api_key
and api_secret
are also needed for secure API calls to Cloudinary (e.g., image and video uploads). You can find your product environment configuration credentials in the API Keys page of the Cloudinary Console.
In addition to the required configuration parameters, you can define a number of optional configuration parameters if relevant.
Setting the configuration parameters can be done globally using either an environment variable or the ObjectUtils.asMap
method, or programmatically in each call to a Cloudinary method. Parameters set in a call to a Cloudinary method override globally set parameters.
secure
configuration parameter is false
. However, for most modern applications, it's recommended to configure the secure
parameter to true
to ensure that your transformation URLs are always generated as HTTPS.Setting the CLOUDINARY_URL environment variable
You can configure the required cloud_name
, api_key
, and api_secret
by defining the CLOUDINARY_URL environment variable. Copy the API environment variable format from the API Keys page of the Cloudinary Console Settings. Replace <your_api_key>
and <your_api_secret>
with your actual values, while your cloud name is already correctly included in the format. When using Cloudinary through a PaaS add-on (e.g., Heroku or AppFog), this environment variable is automatically defined in your deployment environment. For example:
CLOUDINARY_URL=cloudinary://my_key:my_secret@my_cloud_name
Append additional configuration parameters, for example upload_prefix
and secure_distribution
, to the environment variable:
CLOUDINARY_URL=cloudinary://my_key:my_secret@my_cloud_name?secure_distribution=mydomain.com&upload_prefix=myprefix.com
This will enable you to receive a Cloudinary instance:
Cloudinary cloudinary = Singleton.getCloudinary();
Setting configuration parameters globally
Here's an example of setting configuration parameters in your Java application:
import com.cloudinary.*;
...
Cloudinary cloudinary = new Cloudinary(ObjectUtils.asMap(
"cloud_name", "my_cloud_name",
"api_key", "my_api_key",
"api_secret", "my_api_secret",
"secure", true));
Or you can directly register a Cloudinary instance in your initializer code:
SingletonManager manager = new SingletonManager();
manager.setCloudinary(someCloudinaryInstance);
manager.init();
Java capitalization and data type guidelines
When using the Java SDK, keep these guidelines in mind:
- Parameter names:
snake_case
. For example: public_id - Classes:
PascalCase
. For example: CloudinaryImageTag - Methods:
camelCase
. For example: imageUploadTag - Pass parameter data as:
Map
Sample projects
To find additional useful code samples and learn how to integrate Cloudinary with your Java applications, take a look at our Sample Projects. These projects are based on the Spring MVC v3.2.
Photo Album: A fully working web application that allows you to uploads photos, maintain a database with references to them, list them with their metadata, and display them using various cloud-based transformations. Image uploading is performed both from the server side and directly from the browser using a jQuery plugin.
- Learn more about uploading images and videos using the Java library.
- See examples of powerful image and video transformations using Java code, and see our image transformations and video transformation docs.
- Check out Cloudinary's asset management capabilities, for example, renaming and deleting assets, adding tags and metadata to assets, and searching for assets.
- Stay tuned for updates by following the Release Notes and the Cloudinary Blog.