React SDK (Legacy)
Last updated: Apr-23-2025
cloudinary-react
v1.x).For details on migrating to the current version of the SDK (frontend-frameworks
v1.x + js-url-gen
v1.x), see the React SDK migration guide.
Overview
Cloudinary's React SDK provides simple, yet comprehensive image and video upload, transformation, optimization, and delivery capabilities that you can implement using code that integrates seamlessly with your existing React application.
For details on all new features and fixes from previous versions, see the CHANGELOG.
If you haven't already started integrating this SDK, you may want to try the JavaScript js-url-gen library together with the new React frontend-frameworks library.
Quick example
Take a look at the following transformation code and the image it delivers:
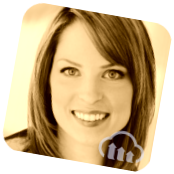
This relatively simple code performs all of the following on the original front_face.jpg image before delivering it:
- Crop to a 150x150 thumbnail using face-detection gravity to automatically determine the location for the crop
- Round the corners with a 20 pixel radius
- Apply a sepia effect
- Overlay the Cloudinary logo on the southeast corner of the image (with a slight offset). The logo is scaled down to a 50 pixel width, with increased brightness and partial transparency (opacity = 60%)
- Rotate the resulting image (including the overlay) by 10 degrees
- Convert and deliver the image in PNG format (the originally uploaded image was a JPG)
And here's the URL that would be included in the image tag that's automatically generated from the above code:
In a similar way, you can transform a video.
- See all possible transformations in the Transformation URL API reference.
- See more examples of image and video transformations using the
cloudinary-react v1.x
library.
React SDK features
- Build URLs for image and video transformation
- Helper elements for embedding and transforming images, and more
Installation and Setup
1. Install the React SDK
The Cloudinary React SDK serves as a layer on top of Cloudinary's JavaScript (cloudinary-core
) library. Install the SDKs by running the following command:
2. Include the required elements of the cloudinary-react library in your code
The following 4 elements are available:
- CloudinaryContext - allows you to define shared parameters that are applied to all child elements.
- Image - defines a Cloudinary Image tag.
- Video - defines a Cloudinary Video tag.
- Transformation - allows you to define additional transformations on the parent element.
3. Set Cloudinary configuration parameters.
To use the Cloudinary React elements, you must configure at least your Cloudinary product environment cloudName
. You can additionally define a number of optional configuration parameters if relevant. You can find your product environment configuration credentials in the API Keys page of the Cloudinary Console Settings. You can apply these settings directly to each element, or you can apply them to all child elements using a CloudinaryContext
element.
- Most functionality implemented on the client side does not require authentication, so only your
cloud_name
is required to be configured, and not your API key or secret. Your API secret should never be exposed on the client side, so if you want to use signed uploads or generate delivery signatures, you'll also need server-side code, for which you can use one of our backend SDKs. - For backward compatibility reasons, the default value of the optional
secure
configuration parameter isfalse
. However, for most modern applications, it's recommended to configure thesecure
parameter totrue
to ensure that your transformation URLs are always generated as HTTPS.
For example:
- Configuration directly in the element:
- Configuration with
CloudinaryContext
applies to all child elements:
Using core Cloudinary JavaScript features
The JavaScript cloudinary-core (legacy) library is the foundation library underlying Cloudinary's React SDK. You can access any of the core JavaScript functionality within your React code after importing the core library. For example:
React capitalization and data type guidelines
When using the React SDK, keep these guidelines in mind:
- Parameter names:
camelCase
. For example: publicId - Classes:
PascalCase
. For example: ImageTag - Methods:
camelCase
. For example: toHTML - Pass parameter data as:
Object
Samples
See our React Photo Album sample project that includes code for uploading files, deleting files, and using dynamic lists: https://github.com/cloudinary/cloudinary-react/tree/master/samples/photo_album
React Storybook tool
You can try out Cloudinary components quickly and easily with our React SDK Storybook, which provides you with a rich visual interface, code playground and inline documentation.
To start using the storybook, select a component from the left-hand menu. Depending on which component you choose, you can either display an asset from your own Cloudinary product environment, experiment by changing parameters, and view the results, or you can scroll and view many different examples of implementations enabled by your selected Cloudinary component.
Either way, when you've found your desired effect, you can copy the code to conveniently implement the results.