Python quick start
Last updated: Apr-09-2024
This quick start lets you get an end-to-end implementation up and running using the Python SDK in 5 minutes or less.
Prerequisites
1. Set up and configure the SDK
In a terminal in your Python3 environment, run the following code:
In your project, create a file called .env
containing your API environment variable from your product environment credentials:
- When writing your own applications, follow your organization's policy on storing secrets and don't expose your API secret.
- Don't store your
.env
under version control for maximum security.
In your project, create a new file called my_file.py
. Copy and paste the following into this file:
2. Upload an image
Copy and paste this into my_file.py
:
3. Get and use details of the image
Copy and paste this into my_file.py
:
4. Transform the image
Copy and paste this into my_file.py
:
5. Run your code
Copy and paste this into my_file.py
:
In the terminal, run the following command:
The following original image is uploaded to Cloudinary, tagged appropriately and accessible via the URL shown below.
The transformed version of the image is accessible via the URL shown below.
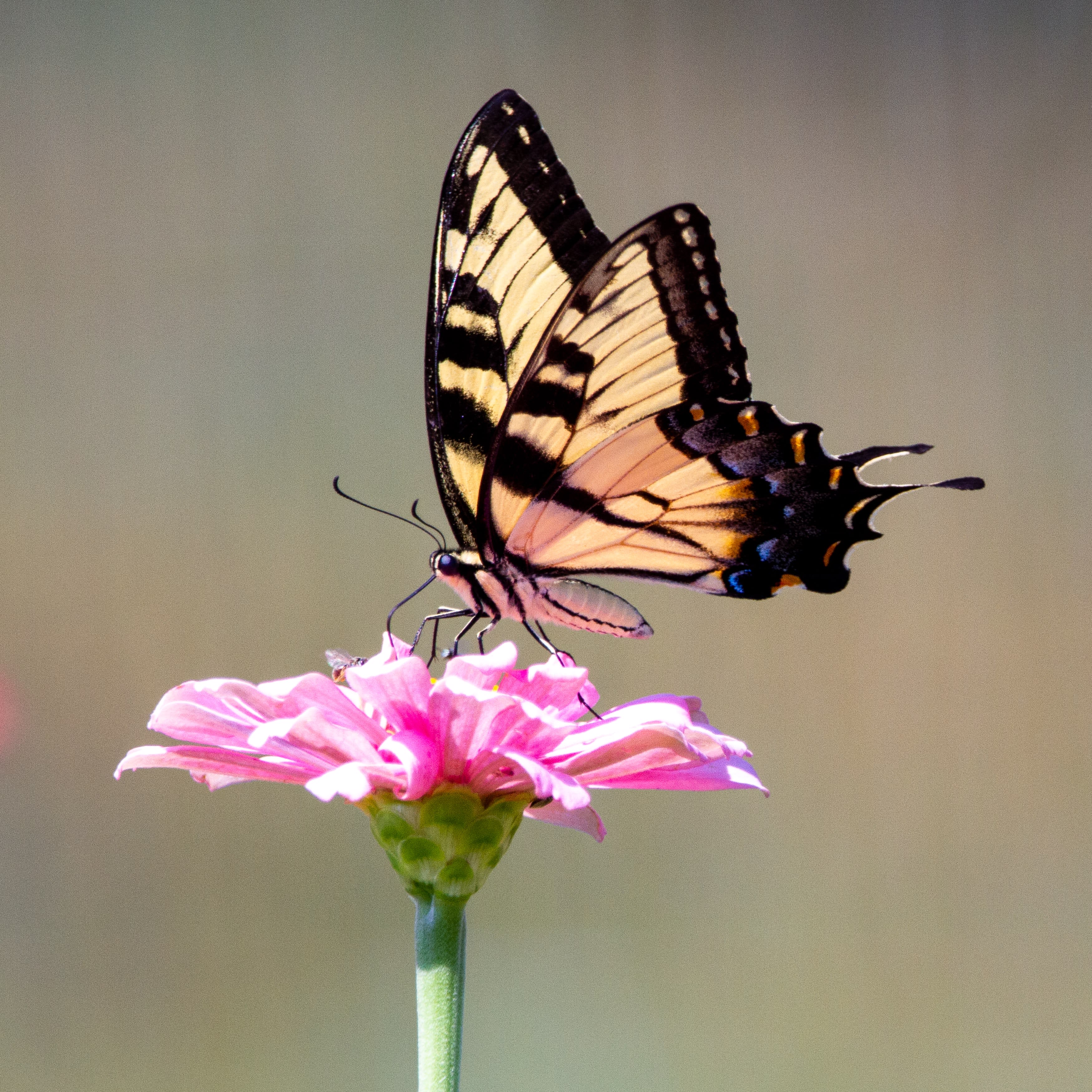
http://res.cloudinary.com/<cloud-name>/image/
upload/v1/quickstart_butterfly
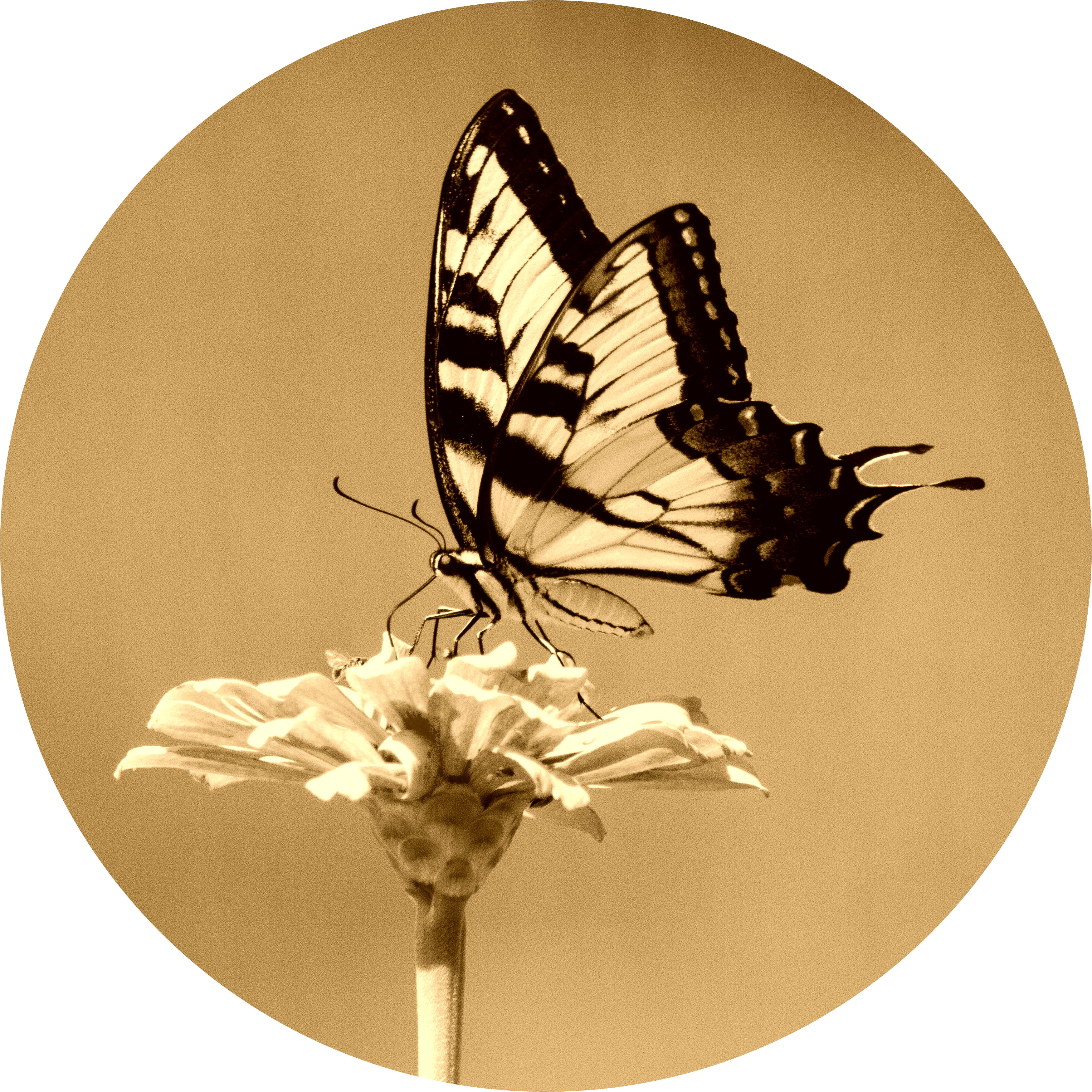
http://res.cloudinary.com/<cloud-name>
/image/upload/e_sepia
r_max/v1/quickstart_butterfly
View the completed code
See the code above in action using this code playground.
- Click Remix to Edit.
- Copy your API environment variable value (i.e., only the portion after the equal sign,
cloudinary://<api_key:api_secret@cloud_name
). - Paste it into the Glitch
.env
file as theVariable Value
forCLOUDINARY_URL
. - Click Logs at the bottom of the screen.
This code is also available in GitHub
Next steps
- Learn more about the Python SDK by visiting the other pages in this SDK guide.
- Get comprehensive details about Cloudinary features and capabilities:
- Upload guide: Provides details and examples of the upload options.
- Image transformations guide: Provides details and examples of the transformations you can apply to image assets.
- Video transformations guide: Provides details and examples of the transformations you can apply to video assets.
- Transformation URL API Reference: Provides details and examples of all available transformation parameters.
- Admin API guide: Provides details and examples of the methods available for managing and organizing your media assets.