Go media transformations
Last updated: Apr-10-2024
After you or your users have uploaded media assets to Cloudinary, you can deliver them via dynamic URLs. You can include instructions in your dynamic URLs that tell Cloudinary to transform your assets using a set of transformation actions. All transformations are performed automatically in the cloud and your transformed assets are automatically optimized before they are routed through a fast CDN to the end user for optimal user experience.
For example, you can resize and crop, add overlay images or concatenate videos, blur or pixelate faces, apply a large variety of special effects and filters, and apply settings to optimize your media and to deliver them responsively.
Cloudinary's Go SDK simplifies the generation of transformation URLs for easy embedding of assets in your Go application.
Syntax overview
The Go SDK allows you to build URLs for delivering assets stored in your Cloudinary product environment, and optionally add transformations by passing them directly as strings. This is enabled by the Go SDK's custom-defined objects which store information about a specified asset.
Using the Go SDK, you can generate a delivery URL:
- Of the original asset.
- With transformations applied.
- With other modifications.
In order to achieve the desired edit or optimization of your asset using the Go SDK, you need to correctly formulate the desired transformation as a string and then pass it as a parameter. For example, if you want to set the height of your asset to 300 pixels and apply a cartoonify effect, you need to pass the string c_scale,w_300/e_cartoonify
as the transformation parameter.
For comprehensive coverage of all available URL transformation parameters and detailed instructions as to how to formulate your transformations, see the Transformation URL API reference
Deliver and transform assets
The @cloudinary/cloudinary-go
library makes it easy for you to create image URLs including any transformation parameters.
Direct URL building
You can build an asset URL by:
-
Instantiating an asset object using
cld.Image(PublicID)
orcld.Video(PublicID)
. - Customize or transform your asset URL by passing parameters to the object.
- Calling the
String()
method of the Go asset object to return the delivery URL.
The resulting URL, myURL
, is:
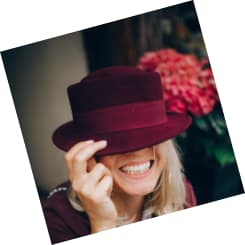
1. Determine the asset type
You can instantiate an object that will capture and provide access to all the information needed to build your asset's delivery URL.
Different object are used to instantiate assets of different types:
Object | Asset type |
---|---|
File |
used for raw assets |
Image |
used for images |
Video |
used for videos |
Media |
used when the asset type is unknown. In this case, the AssetType can be set dynamically. |
Use the appropriate object for your asset type, and identify the asset you are instantiating by passing the object a public ID:
docs/sdk/go
folder.2. Customize and transform your asset delivery URL
The parameters described in the Object parameters table are part of the object you instantiated. These parameters, automatically populated with the values of the asset you identified, contain the information needed to generate the asset's delivery URL:
Object parameters table
Parameter | Description |
---|---|
AssetType | The type of asset (raw , image , or video ), determined by the method you used to create the object (File , Image , or Video ). For the Media object, the default AssetType is image , but you can change this parameter dynamically. |
DeliveryType | Provides an indication about the way the asset will be delivered, although in most cases, the delivery type is determined at the time that the asset is stored in your product environment. The default delivery type is upload . |
Transformation | Contains the transformation string, which, when appended to the delivery URL, programmatically modifies the original image or video asset, resulting in a newly generated (derived) media file based on the original. |
Version | Represents the timestamp of the upload and enables you to access the latest version of an asset, or backed-up versions of it. |
PublicID | The unique identifier of the asset. |
Suffix | A user-friendly, descriptive ending that you can add as a suffix to the public ID in the delivery URL. |
Config | The configuration parameters that you set globally for your SDK. |
You can change parameters stored in the object to modify the delivery URL. For example, you can add a suffix, change the asset type, or change the asset version in the delivery URL.
By assigning a string containing transformation components to the Transformation
parameter, you can change, optimize, edit, and enhance the asset generated by the delivery URL.
Dynamically assign an asset type
To instantiate an object and then dynamically assign it an AssetType:
Change the version of an asset
To instantiate an image object and then change the version of the asset:
Assign a transformation
To instantiate an image object and then assign the Transformation
parameter transformation components:
In the above example:
* f_auto
and q_auto
optimize the format and quality of the image automatically
* c_fill
crops the image while keeping its aspect ratio
* g_face
keeps the image focused on the face after cropping
* w_250
and h_250
set the width and height of the transformed image to 250px
* e.sepia
applies a special effect called "sepia" to the image.
3. Return the delivery URL
If you added a transformation or modified the parameters of the object, the URL you generate will reflect those changes.
The following code generates the URL from the example above:
The resulting URL and image:
Combining transformations
Cloudinary supports powerful transformations. You can even combine multiple transformations together as part of a single transformation request, e.g. crop an image and add a border. In certain cases you may want to perform additional transformations on the result of the previous transformation request.
To support multiple transformations in a transformation URL, you can include multiple transformation components, each separated by a '/'. Each transformation component is applied to the result of the previous one. In Go, applying multiple transformations is achieved by simply adding the next transformation to your transformation string, and then assigning that value to the Transformation parameter of your asset object. The following example first crops the original image to a specific set of custom coordinates and then transforms the result so it fills a 130x100 rectangle:
Apply common image transformations
This section provides an overview and examples of the following commonly used image transformation features, along with links to more detailed documentation on these features:
- Resizing and cropping
- Converting to another image format
- Applying image effects and filters
- Adding text and image overlays
- Image optimizations
Keep in mind that this section is only intended to introduce you to the basics of using image transformations with Go.
For comprehensive explanations of how to implement a wide variety of transformations, see Image transformations.
For a full list of all supported image transformations and their usage, see the Transformation URL API Reference.
Resizing and cropping
There are a variety of different ways to resize and/or crop your images, and to control the area of the image that is preserved during a crop.
The following example shows an image cropped (c_fill) to a 350X350 square (w_350,h_350) while keeping focus on the faces (g_faces) in the image:
You can also use automatic gravity to determine what to keep in the crop automatically.
For all the ways in which an image can be cropped, see the parameters under the c (crop) section of the Transformation URL Reference.
For details on all resizing and cropping options, see Image resizing and cropping.
Converting to another image format
You can deliver any image uploaded to Cloudinary in essentially any image format. There are three ways to convert and deliver images in another format:
- Specify the image's public ID with the desired extension.
- Explicitly set the desired format using the
Transformations
parameter. - Use the
auto
fetch_format to instruct Cloudinary to deliver the image in the most optimized format for each browser that requests it.
For example:
Deliver a .jpg file in .png format:
Let Cloudinary select the optimal format for each browser:
For example, in Chrome, this image may deliver in .avif or .webp format (depending on your product environment setup):
The above code generates a URL with the f_auto
parameter:
For information about the relevant parameter, see the f (format) section of the Transformation URL Reference.
For more details, see:
- Delivering images in different formats
- Automatic format selection (f_auto)
- Tips and considerations for using f_auto
Applying image effects and filters
You can select from a large variety of image effects, enhancements, and filters to apply to your images. The available effects include various color balance and level effects, tinting, blurring, pixelating, sharpening, automatic improvement effects, artistic filters, image and text overlays, distortion and shape changing effects, outlines, backgrounds, shadows, and more.
For example, the code below applies a cartoonify effect (e_cartoonify), rounding corners effect (r_max), and background color effect (b_lightblue), and then crops and pads (c_pad) the image down to a height of 300 pixels.
For all the effects that can be applied to images, see the parameters under the e (effect) section of the Transformation URL Reference.
For more details on the available image effects and filters, see Visual image effects and enhancements.
Adding text and image overlays
You can add images and text as overlays on your main image. You can apply the same types of transformations on your overlay images as you can with any image and you can use gravity settings or x and y coordinates to control the location of the overlays. You can also apply a variety of transformations on text, such as color, font, size, rotation, and more.
For example, the code below overlays a couple's photo on a mug image (l_nice_couple). The overlay photo is cropped using face detection (g_faces) with adjusted color saturation (e_saturation:50) and a vignette effect (e_vignette) applied. The word love is added in a brown, fancy font (l_text:Cookie_23_bold:Love,co_rgb:744700) and placed to fit the design (x_-23,y_50). Additionally, the final image is cropped (c_crop,w_300,h_250,x_30) and the corners are rounded (r_60).
For all ways in which you can apply overlays to images, see the parameters under the l (layer) section of the Transformation URL Reference.
For more details on adding image overlays, see Visual image effects and enhancements.
Image optimizations
By default, Cloudinary automatically performs certain optimizations on all transformed images. There are also a number of additional features that enable you to further optimize the images you use in your application. These include optimizations to image quality, format, and size, among others.
For example, you can use the auto
value for the fetch_format and quality attributes to automatically deliver the image in the format and quality that minimize file size while meeting the required quality level. In addition, resize the image to make it even lighter. Below, these parameters are applied, resulting in a 25.66 KB AVIF file (in Chrome) instead of a 1.34 MB JPG with no visible change in quality.
For all ways in which you can apply overlays to images, see the parameters under the q (quality) and f (format) sections of the Transformation URL Reference.
For an in-depth review of the many ways you can optimize your images, see Image optimization.
Apply common video transformations
This section provides an overview and examples of the following commonly used image transformation features, along with links to more detailed documentation on these features:
- Resizing and cropping videos
- Concatenating videos
- Trimming videos
- Adding video overlays
- Adding video effects
Keep in mind that this section is only intended to introduce you to the basics of using video transformations with Go.
For a full list of all supported video transformations and their usage, see the Transformation URL API Reference.
For comprehensive explanations of how to implement a wide variety of transformations, see Video transformations.
Resizing and cropping videos
There are a variety of different ways to resize and/or crop your videos, and to control the area of the video that is preserved during a crop. The following examples:
- crop the video to a specified dimension (c_crop,h_800,w_750) in a specified area (x_200,y_450)
- resize the video to fit a specified height and width while padding the extra space (c_pad,h_320,w_480) with the original asset blurred playing in the background (b_blurred:400:15) to preserve the original aspect ratio.
c_crop,x_200,y_450
Pad with blurred videoc_pad,b_blurred:380:15
For all the ways in which a video can be cropped, see the parameters under the c (crop) section of the Transformation URL Reference that are marked as supported for video.
For details on all resizing and cropping options, see Resizing and cropping videos.
Concatenating videos
You can concatenate videos with a variety of options, including concatenating only a portion of the videos, concatenating a video at the beginning or end of another, creating custom transitions between concatenated videos, and concatenating an image to a video.
The following example shows two videos shortened to 5 seconds each (du_5), the main video starting with an offset of 1 second (so_1), with both videos uniformly resized (c_fill,h_200,w_300) and concatenated (fl_splice):
For a detailed description of how to concatenate videos, see the splice parameter of the fl_flag section of the Transformation URL Reference.
For details on all the ways in which you can concatenate videos, see Concatenating media.
Trimming videos
When trimming your videos, you can determine when to start trimming, when to stop, and / or the duration of the trimmed video.
The following example shows trimming a video to the section that starts at 3.5 seconds (so_3.5) with a duration of 5 seconds (du_5):
For all the ways in which a video can be trimmed, see the so (start_offset), du (duration), eo (end_offset) parameters the Transformation URL Reference that are marked as supported for video.
For more details on trimming videos, see Trimming videos.
Adding video overlays
You can add video, text, or image overlays onto your videos.
The following example shows one video scaled down (c_scale,w_100) and placed in the north-east corner (g_north_east) as an overlay (l_video:exercise2), starting 3 seconds after the main video starts playing (so_3):
For all the ways in which you can add video overlays, see the parameters under the l (layer) section of the Transformation URL Reference that are marked as supported for video.
For more details on video overlays, see Placing layers on videos.
Adding video effects
You can select from a large variety of video effects, enhancements, and filters to apply to your video. The available effects include speed, direction, and looping control, adding a progress indicator overlay, different transparency settings, AI generated preview, a variety of color balance and level effects, blurring, fading, and automatic improvement effects.
The following example shows a video that fades in and out (e_fade:2000/e_fade:-4000), loops twice (e_loop:2), and has a vignette filter applied (e_vignette:50):
For all video effects, see the parameters under the e (effect) section of the Transformation URL Reference that are marked as supported for video.
For an in-depth review of all the available video effects, see Video Effects.
Try it out!
Here's the code in a fully functioning Go app. It's structured a little differently from above - each transformation example has its own Go file showing the imports and syntax required. Find those files in the go/src/lib
folder of the project.
main.go
imports all the functions to display the transformed images on a simple web page.
Experiment with images:
This code is also available in GitHub.
Experiment with videos:
This code is also available in GitHub
- Learn more about uploading images and videos using the Go SDK.
- See examples of powerful image and video transformations using, and see our image transformations and video transformations docs.
- Check out Cloudinary's asset management capabilities.
- Stay tuned for updates with the Programmable Media Release Notes and the Cloudinary Blog.